binary tree python insert
The Binary search tree can be empty. What is the height of a binary tree.
Let min min d and max - d max where d max-min2 be the left and right child respectively.
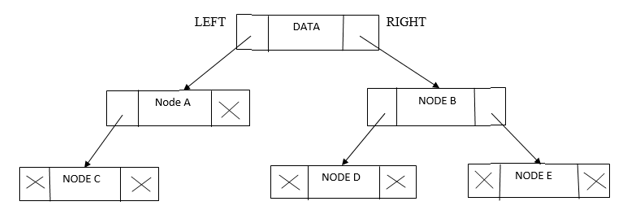
. Read on to see how you can write an implementation of binary search tree in Python from scratch. To insert into a tree we use the same node class created above and add a insert class to it. Def __init__ self key function to insert data to our binary tree selfleftchild None setting leftchild of the tree to add items selfrightchild None setting rightchild of the tree.
Please try your approach on IDE first before moving on to the solution. The idea is to do an iterative level order traversal of the given tree using queue. Finally the PrintTree class is used to print the tree.
Algorithm Binary Search Trees Binary Search Tree - Insertion Python Example This is a simple implementation of Binary Search Tree Insertion using Python. The Value to be inserted is less than the root. We need the following Python syntax to generate a binary tree.
Implement binary search tree insert function Creating a class for node object class Node object. Finally the PrintTree method is used to print the tree. The binary tree is comprised of nodes and these nodes each being a data component have left and right child nodes.
Binary trees are useful for storing data in an organized manner so that it can be quickly retrieved inserted updated and deleted. Given a binary tree and a key insert the key into the binary tree at the first position available in level order. To be precise binary search trees provide an average Big-O complexity of Ologn for search insert update.
While inserting a node in a binary search tree three conditions may arise. An example is shown below. Let min max be the root node.
Algorithm for Binary Tree Insertion When a node is inserted in Binary Tree the new node always checks with its parent node. Then it has 100 points. The insert class compares the value of the node to the parent node and decides to add it as a left node or a right node.
Root itself will be a value None. If the new node is less than the value of parent node the new node will be placed on the left side of the parent otherwise the. A binary tree in Python is a nonlinear data structure used for data search and organization.
The value to be inserted is greater than the root. Selfleft None selfright None selfdata None. To add an element into a binary tree we just need to write the insert function compares the value of the node to the parent node and decides to add it as a left node or a right node.
The code below shows the insertion function which places the node in a tree such that none of the properties of binary search tree is violated. Following the code snippet each image shows the execution visualization which makes it easier to visualize how this code works. To implement the first condition we just make a new node and declare it as root.
A recursive function is required since the sub-tree has similar elements. Initializing to None def __init__ self. Import numpy as np dataset nprandomnormal 5010100 Record the min and max for this dataset.
Get to know about balanced binary tree full binary tree and complete binary tree.
Binary Search Tree Implementation 101 Computing
Binary Tree Basic Introduction And Code Implementation To Create Tree In Python Youtube
Python Binary Tree Implementation Python Guides
Binary Tree Methods In Python Kevin Vecmanis
Invert A Binary Tree Python Code With Example Favtutor
Binary Search Tree Bst Complete Implementation In Java Tutorialhorizon
Height Of A Binary Tree Python Code With Example Favtutor
Binary Tree In Python What A Binary Tree Is With Examples
How Do We Get A Balanced Binary Tree By Jake Zhang The Startup Medium
Ways To Traverse A Binary Tree Using The Recursive And Iterative Approach By Wangyy Medium
Height Of A Binary Tree Python Code With Example Favtutor
Relationship Between Number Of Nodes And Height Of Binary Tree Geeksforgeeks
Build The Forest In Python Series Binary Tree Traversal Shun S Vineyard
Insertion In A Binary Tree In Level Order Geeksforgeeks
Level Order Traversal Of Binary Tree Python Code Favtutor